This Tutorial site will help to learn Java ,JavaScript, Computer Science for beginners. we also covered bonus topics like Finance, Health and Hindu | Indian boys and girls name .
Sunday, January 9, 2022
How to make JavaScript projects or app?
Friday, December 25, 2020
Lotto App|Random Number App|Javascript app
Random Number App: In this tutorial you will make app similar to Lottery or pick 3 number app. It is fun way to make app . You will use HTML, CSS and JavaScript . In JavaScript you will use Random generator and DOM (Document Object Model). How these topics go to gather and by using Event what you make. Here is source code and video for it.
Video:
Source Code:
Saturday, November 21, 2020
How to create simple Quiz app in Javascript?
Quiz App in Javascript:
In this tutorial you will learn HTML, CSS, BootStrap and Java library -Jquery. This is fun way to learn these topics by making app.You will learn how to use show, hide and toggle function by making cool app. Video and Source code are included so make and show to world.
Video:
SourceCode:
Easy Javascript App for beginner | Feet to Inch App
JavaScript App for Beginner: In this tutorial you will learn HTML, CSS, Bootstrap, JavaScript function, event, DOM, Validation , if else statement and many more by creating app . It is learn with fun activity . you will learn how to create Feet to Inch conversion. so make it and show to world. Below you will find video and source code for App.
lets understand one by one. First lets create HTML Document
h1tag contains title, now add two input tag and set type as text . now we will add one button . That's what we need for html . now we since we will add CSS in future so lets wrap all html in div and give id as container. we will add id to input tag also . For error message lets give h3 tag and add span tag for future error message. for coloring button we will give id to button tag also.
now we can create style tag after title in html.
we can do this multiple way . best way is do it via creating separate file and link it.
for Simplicity purpose we have created style tag in same file . Since we gave id
for container so we will use hashtag for container and we will set height , width color
etc. Now our CSS is also ready .
Since we have added our submit butoon in variable we can add even listener on it. so we
create function when someone click on button our function will run. we will add if else
statement . if value of feet is empty, we will through error message and we have provide
error message text as "Invalid, can not empty!". If user enter any content in input field
it will run else block of code. we will clear error message first. and will provide
formula which will convert value accurately.
For more information we have added video tutorial step by step so you can watch it also.
in this video tutorial you will learn about HTML, CSS, Bootstrap. JavaScript is very important
we have covered datatype variable how to use it, Function, if else statement, Document Object Model (DOM)
by adding and fetching id and store in variable. now you can create many app just need to change formula accordingly .
Video
Source-code
how to create Simple Javascript App?
LB to KG Calculator
LB: KG:
Sunday, June 14, 2020
JavaScript Projects for Beginners
Javascript for Beginners sourceCode-
1. Print 0 to 10 by using for loop and while loop
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function number(){
// for(let i= 1;i<=10;i++){
// document.write("the nember is "+i+"<br>");
// }
let i=1;
while(i<=10){
document.write(`the new while number is ${i} <br> `);
i++;
}
}
number();
</script>
</body>
</html>
2. How to Print number in descending order in javascript?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function dec(){
for(let i = 10; i>=1;i--){
document.write(`The number is ${i}<br>`)
}
}
dec();
</script>
</body>
</html>
3. Print Odd Number
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function odd(){
for(let i = 1;i<=10;i+=2){
document.write(`Method one with for loop ${i} <br>`);
}
for(let k=1;k<=10;k++) {
if(k%2!==0){
document.write(`Method two with for loop ${k} <br>`);
}
}
//with while loop
let j = 1;
while(j<=10) {
document.write(`Method one with while loop ${j} <br>`);
j+=2;
}
// print 1 to 10 only even number by mod method with for loop
}
odd();
</script>
</body>
</html>
4. Print Even Number
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
//50 -100 even
function even(){
for(let i= 50;i<=100;i++){
if(i%2==0){
document.write(`Then even number is ${i}<br>`)
}
}
}
even();
</script>
</body>
</html>
5. Print table of 3 by using for loop
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function tab(){
let j= 3
for(let i= 1;i<=10;i++){
document.write(`${j} times ${i} is ${j*i}<br>`)
}
}
tab();
</script>
</body>
</html>
6. How to add or Sum in Javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function total(){
sum=0
let array=[5,3,2,5,1]
for(let i=0;i<array.length;i++){
sum+=array[i]
}
document.write(`Total is ${sum}`);
}
total();
</script>
</body>
</html>
7. Find smallest or Min number in Array in Javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function minimum(){
let array= [5,10,15,2,20,4]
let min=array[0]
for(let i = 0 ;i<array.length;i++){
if(min>array[i]){
min= array[i]
}
}
document.write(`The minimum value is ${min}`)
}
minimum();
</script>
</body>
</html>
8. Find Largest or Max number in Javascript Array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function maximum(){
let array = [5,4,2,6,10,1]
let max= array[0]
for(let i=0;i<array.length;i++){
if(array[i]>max){
max= array[i]
}
}
document.write(`The largest element in array is ${max}`)
}
maximum();
</script>
</body>
</html>
9. Find Average in Javascript Array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function avg(){
let sum= 0;
let array= [1,2,3]
for(let i= 0;i<array.length;i++){
sum+=array[i]
}
document.write(`The average is ${sum/array.length}`)
}
avg();
</script>
</body>
</html>
java switch statement | Java Tutorial For Beginners
Switch statement is alternative and more cleaner way to write code if we have more than two options. we can use else if, this is better way...
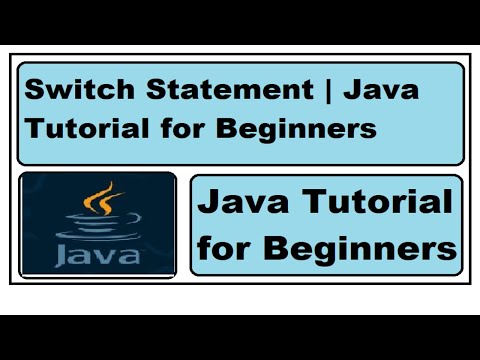
-
**Privacy Policy** Guruji Guru built the General Knowledge Quiz app as a Free app. This SERVICE is provided by Guruji Guru at no cost and is...
-
Javascript for Beginners sourceCode- 1. Print 0 to 10 by using for loop and while loop IF you want to print 0 to 1o in descending order...
-
Random Number App: In this tutorial you will make app similar to Lottery or pick 3 number app. It is fun way to make app . You will use HT...